TL; DR: my simple Python code to output a result of text comparison in git-diff style
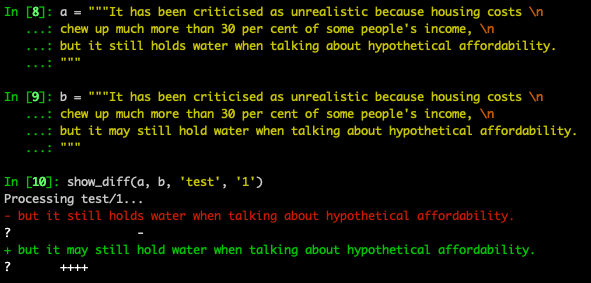
Here’s the code snippet in Python.
from difflib import Differ from termcolor import colored def color_print(buffer, line): # skip if the line isn't changed if line.startswith(' '): return # otherwise render deleted line in red and new line in green if line.startswith('-'): color = 'red' elif line.startswith('+'): color = 'green' else: color = 'white' buffer.append(colored(line, color)) def show_diff(live, revision, domain, rule): d = Differ() results = [] # split origin text into a list of strings, then use Differ().compare() for line in d.compare(live.splitlines(keepends=True), revision.splitlines(keepends=True)): color_print(results, line) print(f'Processing {domain}/{rule}...') sys.stdout.writelines(results) print()
🙂